ProgressBar is a graphical presentation which shows some progress. It shows a bar representing the completing of the task. Normally the ProgresBar do not display the amount of completion in numbers. If we want, we can display it in the Text or as graphical format.
In this tutorial we are going to explain how we can make a simple and stylish progress bar using jQuery and HTML/css. Here we are explaining five types of progress.
You can also refer our live demo or download the Script file. Extract the downloaded files, save it and run index.html on browser
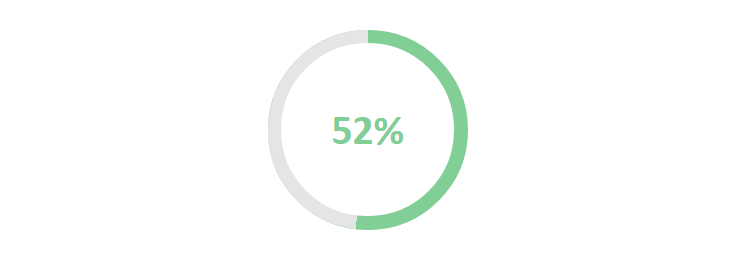
HTML Code
Copy below code in your index.html page.
<div class="bar_container">
<div id="main_container">
<div id="pbar" class="progress-pie-chart" data-percent="0">
<div class="ppc-progress">
<div class="ppc-progress-fill"></div>
</div>
<div class="ppc-percents">
<div class="pcc-percents-wrapper">
<span>%</span>
</div>
</div>
</div>
<progress style="display: none" id="progress_bar" value="0" max="100"></progress>
</div>
</div>
CSS code
Copy below code in your style.css file or use internal, inside style tag.
/* Pie Chart */
.progress-pie-chart {
width:200px;
height: 200px;
border-radius: 50%;
background-color: #E5E5E5;
position: relative;
}
.progress-pie-chart.gt-50 {
background-color: #81CE97;
}
.ppc-progress {
content: "";
position: absolute;
border-radius: 50%;
left: calc(50% - 100px);
top: calc(50% - 100px);
width: 200px;
height: 200px;
clip: rect(0, 200px, 200px, 100px);
}
.ppc-progress .ppc-progress-fill {
content: "";
position: absolute;
border-radius: 50%;
left: calc(50% - 100px);
top: calc(50% - 100px);
width: 200px;
height: 200px;
clip: rect(0, 100px, 200px, 0);
background: #81CE97;
transform: rotate(60deg);
}
.gt-50 .ppc-progress {
clip: rect(0, 100px, 200px, 0);
}
.gt-50 .ppc-progress .ppc-progress-fill {
clip: rect(0, 200px, 200px, 100px);
background: #E5E5E5;
}
.ppc-percents {
content: "";
position: absolute;
border-radius: 50%;
left: calc(50% - 173.91304px/2);
top: calc(50% - 173.91304px/2);
width: 173.91304px;
height: 173.91304px;
background: #fff;
text-align: center;
display: table;
}
.ppc-percents span {
display: block;
font-size: 2.6em;
font-weight: bold;
color: #81CE97;
}
.pcc-percents-wrapper {
display: table-cell;
vertical-align: middle;
}
.progress-pie-chart {
margin: 50px auto 0;
}</style>
jQuery Code
Copy below script in your script.js file or use internal, inside script tag.
$(document).ready(function() {
var progressbar = $('#progress_bar');
max = progressbar.attr('max');
time = (1000 / max) * 5;
value = progressbar.val();
var loading = function() {
value += 1;
addValue = progressbar.val(value);
$('.progress-value').html(value + '%');
var $ppc = $('.progress-pie-chart'),
deg = 360 * value / 100;
if (value > 50) {
$ppc.addClass('gt-50');
}
$('.ppc-progress-fill').css('transform', 'rotate(' + deg + 'deg)');
$('.ppc-percents span').html(value + '%');
if (value == max) {
clearInterval(animate);
}
};
var animate = setInterval(function() {
loading();
}, time);
});
HTML Code
Copy below code in your index.html page.
<div class="progress">
<div class="circle done">
<span class="label">1</span>
<span class="title">0%</span>
</div>
<span class="bar done"></span>
<div class="circle done">
<span class="label">2</span>
<span class="title">25%</span>
</div>
<span class="bar half"></span>
<div class="circle active">
<span class="label">3</span>
<span class="title">50%</span>
</div>
<span class="bar"></span>
<div class="circle">
<span class="label">4</span>
<span class="title">75%</span>
</div>
<span class="bar"></span>
<div class="circle">
<span class="label">5</span>
<span class="title">100%</span>
</div>
</div>
CSS Code
Copy below code in your style.css file or use internal, inside style tag.
.progress {
width: 1000px;
margin: 20px auto;
text-align: center;
}
.progress .circle,
.progress .bar {
display: inline-block;
background: #fff;
width: 40px; height: 40px;
border-radius: 40px;
border: 1px solid #d5d5da;
}
.progress .bar {
position: relative;
width: 80px;
height: 6px;
top: -33px;
margin-left: -5px;
margin-right: -5px;
border-left: none;
border-right: none;
border-radius: 0;
}
.progress .circle .label {
display: inline-block;
width: 32px;
height: 32px;
line-height: 32px;
border-radius: 32px;
margin-top: 3px;
color: #b5b5ba;
font-size: 17px;
}
.progress .circle .title {
color: #b5b5ba;
font-size: 13px;
line-height: 30px;
margin-left: -5px;
}
/* Done / Active */
.progress .bar.done,
.progress .circle.done {
background: #eee;
}
.progress .bar.active {
background: linear-gradient(to right, #EEE 40%, #FFF 60%);
}
.progress .circle.done .label {
color: #FFF;
background: #81CE97;
box-shadow: inset 0 0 2px rgba(0,0,0,.2);
}
.progress .circle.done .title {
color: #444;
}
.progress .circle.active .label {
color: #FFF;
background: #0c95be;
box-shadow: inset 0 0 2px rgba(0,0,0,.2);
}
.progress .circle.active .title {
color: #0c95be;
}
jQuery Code
Copy below script in your script.js file or use internal, inside script tag.
$(document).ready(function() {
var i = 1;
$('.progress .circle').removeClass().addClass('circle');
$('.progress .bar').removeClass().addClass('bar');
setInterval(function() {
$('.progress .circle:nth-of-type(' + i + ')').addClass('active');
$('.progress .circle:nth-of-type(' + (i - 1) + ')').removeClass('active').addClass('done');
$('.progress .circle:nth-of-type(' + (i - 1) + ') .label').html('✓');
$('.progress .bar:nth-of-type(' + (i - 1) + ')').addClass('active');
$('.progress .bar:nth-of-type(' + (i - 2) + ')').removeClass('active').addClass('done');
i++;
if (i == 0) {
$('.progress .bar').removeClass().addClass('bar');
$('.progress div.circle').removeClass().addClass('circle');
i = 1;
}
}, 1000);
});
HTML Code
Copy below code in your index.html page.
<div class="p_bar_body"><progress id="progress_bar" value="0" max="100"></progress><div class="progress-value"></div></div>
CSS Code
Copy below code in your style.css file or use internal, inside style tag.
progress {
width: 500px;
height: 25px;
border: 0px none;
background-color: #E5E5E5;
border-radius: 10px;
padding: 4px 5px 5px 5px;
}
progress::-webkit-progress-bar {
background-color: #E5E5E5;
border-radius: 50px;
padding: 2px;
box-shadow: 0 1px 0px 0 rgba(255, 255, 255, 0.2);
}
progress::-webkit-progress-value {
border-radius: 50px;
background:
-webkit-linear-gradient(135deg, transparent, transparent 20%, rgba(0, 0, 0, 0.1) 33%, #45B565 50%, transparent 10%),
-webkit-linear-gradient(top, #81CE97, #81CE97),
-webkit-linear-gradient(left, #ba7448, #c4672d);
background-size: 25px 14px, 100% 100%, 100% 100%;
-webkit-animation: move 5s linear 0 infinite;
}
@-webkit-keyframes move {
0% {background-position: 0px 0px, 0 0, 0 0}
100% {background-position:100px 0px, 0 0, 0 0}
}
.progress-value{
color: #444;
margin-left: 507px;
margin-top: -24px;
}
.progressDiv {
width: 84%;
background: #fcfcfc;
height: 325px;
border: 1px solid #ccc;
position: relative;
left: 7%;
top: 100px;
display: inline-block;
border-radius: 2px;
box-shadow: 0px 1px 1px 1px #ccc;
}
jQuery Code
Copy below script in your script.js file or use internal, inside script tag.
$(document).ready(function() {
var progressbar = $('#progress_bar');
max = progressbar.attr('max');
time = (1000 / max) * 5;
value = progressbar.val();
var loading = function() {
value += 1;
addValue = progressbar.val(value);
$('.progress-value').html(value + '%');
if (value == max) {
clearInterval(animate);
}
};
var animate = setInterval(function() {
loading();
}, time);
});
HTML Code
Copy below code in your index.html page.
<div class="container">
<ul id="progress">
<li><div id="layer1" class="ball"></div></li>
<li><div id="layer2" class="ball"></div></li>
<li><div id="layer3" class="ball"></div></li>
<li><div id="layer4" class="ball"></div></li>
<li><div id="layer5" class="ball"></div></li>
</ul>
</div>
CSS Code
Copy below code in your style.css file or use internal, inside style tag.
ul#progress {
list-style:none;
width:125px;
margin:0 auto;
padding-top:50px;
padding-bottom:50px;
padding-right: 46px;
}
ul#progress li {
float:left;
position:relative;
width:15px;
height:15px;
border:1px solid #fff;
border-radius:50px;
margin-left:10px;
border-left:1px solid #E5E5E5; border-top:1px solid #E5E5E5; border-right:1px solid #E5E5E5; border-bottom:1px solid #E5E5E5;
background:#E5E5E5;;
}
ul#progress li:first-child { margin-left:0; }
.running .ball {
background-color:#b5b5ba;
background-image: -moz-linear-gradient(90deg, #2187e7 25%, #a0eaff);
background-color:#81CE97;
width:15px;
height:15px;
border-radius:50px;
-moz-transform:scale(0);
-webkit-transform:scale(0);
-moz-animation:loading 1s linear forwards;
-webkit-animation:loading 1s linear forwards;
}
#layer1 { -moz-animation-delay:0.5s; -webkit-animation-delay:0.5s; }
#layer2 { -moz-animation-delay:1s; -webkit-animation-delay:1.5s; }
#layer3 { -moz-animation-delay:1.5s; -webkit-animation-delay:2.5s; }
#layer4 { -moz-animation-delay:2s; -webkit-animation-delay:3.5s; }
#layer5 { -moz-animation-delay:2.5s; -webkit-animation-delay:5s; }
@-moz-keyframes loading {
0%{-moz-transform:scale(0,0);}
100%{-moz-transform:scale(1,1);}
}
@-webkit-keyframes loading {
0%{-webkit-transform:scale(0,0);}
100%{-webkit-transform:scale(1,1);}
}
jQuery Code
Copy below script in your script.js file or use internal, inside script tag.
$(document).ready(function() {
$('#progress').removeClass('running');
$('#progress').removeClass('running').delay(10).queue(function(next) {
$(this).addClass('running');
next();
});
return false;
});
HTML Code
Copy below code in your index.html page.
<div class="container">
<ul id="loadbar">
<li><div id="layerFill1" class="bar"></div></li>
<li><div id="layerFill2" class="bar"></div></li>
<li><div id="layerFill3" class="bar"></div></li>
<li><div id="layerFill4" class="bar"></div></li>
<li><div id="layerFill5" class="bar"></div></li>
<li><div id="layerFill6" class="bar"></div></li>
<li><div id="layerFill7" class="bar"></div></li>
<li><div id="layerFill8" class="bar"></div></li>
<li><div id="layerFill9" class="bar"></div></li>
<li><div id="layerFill10" class="bar"></div></li>
</ul>
</div>
CSS Code
Copy below code in your style.css file or use internal, inside style tag.
ul#loadbar {
list-style:none;
width:140px;
margin:0 auto;
padding-top:50px;
padding-bottom:75px;
padding-right: 46px;
}
ul#loadbar li {
float:left;
position:relative;
width:11px;
height:26px;
margin-left:1px;
border-left:1px solid #E5E5E5; border-top:1px solid #E5E5E5; border-right:1px solid #E5E5E5; border-bottom:1px solid #E5E5E5;
background:#E5E5E5;
}
ul#loadbar li:first-child { margin-left:0; }
.ins .bar {
background-color:#81CE97;
width:11px;
height:26px;
opacity:0;
-webkit-animation:fill .5s linear forwards;
-moz-animation:fill .5s linear forwards;
}
#layerFill1 { -moz-animation-delay:0.5s; -webkit-animation-delay:0.5s; }
#layerFill2 { -moz-animation-delay:1s; -webkit-animation-delay:1s; }
#layerFill3 { -moz-animation-delay:1.5s; -webkit-animation-delay:1.5s; }
#layerFill4 { -moz-animation-delay:2s; -webkit-animation-delay:2s; }
#layerFill5 { -moz-animation-delay:2.5s; -webkit-animation-delay:2.5s; }
#layerFill6 { -moz-animation-delay:3s; -webkit-animation-delay:3s; }
#layerFill7 { -moz-animation-delay:3.5s; -webkit-animation-delay:3.5s; }
#layerFill8 { -moz-animation-delay:4s; -webkit-animation-delay:4s; }
#layerFill9 { -moz-animation-delay:4.5s; -webkit-animation-delay:4.5s; }
#layerFill10 { -moz-animation-delay:5s; -webkit-animation-delay:5s; }
@-moz-keyframes fill {
0%{ opacity:0; }
100%{ opacity:1; }
}
@-webkit-keyframes fill {
0%{ opacity:0; }
100%{ opacity:1; }
}
jQuer Code
Copy below script in your script.js file or use internal, inside script tag.
$(document).ready(function() {
$('#loadbar').removeClass('ins');
$('#loadbar').removeClass('ins').delay(10).queue(function(next) {
$(this).addClass('ins');
next();
});
return false;
});
Conclusion:
In this tutorial we have learned about, how we can make different types of progress bar. Hope you have benefited from it. Keep visiting our websites for more knowledge and information.
You may also like some related post –
- CSS list – Design stylish list
- CSS Radio Button And Checkbox
4 Replies to “jQuery CSS Progress Bar”
Neeraj,
I’m trying to use Steps Progress Bar… That one is amazing. Very useful. Clean!!!
Thanks for your tutorials. Great job.
This website is incredible. I found the examples very important in my career. thank you guys
How to i change Circular Progress Bar size i want to reduce it’s size
Hi! Dami,
What I did… I just played with its smart css.